Explain the purpose of the Link component in React Router.
Last Updated :
27 Feb, 2024
React Router is a library in the React JS application. It is used to navigate the page to another page. It allows developers to create a seamless and dynamic user experience. It has many features the ‘Link’ Component stands out as a powerful tool for creating navigation links in React Applications.
What is a Link Component?
The ‘Link’ component is a part of the React Router Library, which is used for handling navigation in React Applications. The purpose of the ‘Link’ component is to create a clickable link that allows users to navigate between different web pages or components.
Syntax of Link Component:
import { Link } from 'react-router-dom';
<Link to="/path">Link Text</Link>
Difference between <a> and Link?
We already know that our JSX also supports the ‘<a>’ anchor tag in React Js, But why use the Link component instead of an anchor tag? Let’s understand the key difference between those.
‘<a>’ Tag
|
‘Link’ Component
|
It triggers full page reload
|
It performs navigation without reloading the page
|
It is standard html tag
|
It is designed specifically for React Application
|
It requiest event handler for prevent its default behaviour
|
It does not requre any event handler
|
It has standard html features
|
It is in built React Router library
|
Basic Usage of Link Component
Using the ‘Link’ Component is easy. First make sure you have install ‘react-router-dom’ in your React Application. After installing the react-router-dom. Import the ‘Link’ Component from the ‘react-router-dom’ and use it to wrap around elements that should trigger navigation.
npm i react-router-dom
<Link to ="/home"> Home </Link>
Example: This example demonstrates the use of React Js Link component to naviagte one page to another Page and back to the first page.
Javascript
import { BrowserRouter, Routes, Route } from "react-router-dom" ;
import FirstPage from "./components/FirstPage" ;
import SecondPage from "./components/SecondPage" ;
function App() {
return (
<BrowserRouter>
<Routes>
<Route path= '/' element={<FirstPage />} />
<Route path= '/second' element={<SecondPage />} />
</Routes>
</BrowserRouter>
);
}
export default App;
|
Javascript
import { Link } from "react-router-dom"
export default function FirstPage() {
return (
<>
<h2> Hey, I am first Page</h2>
<Link to= "/second" >Click for Second Page</Link>
</>
)
}
|
Javascript
import { Link } from "react-router-dom" ;
export default function SecondPage() {
return (
<div>
<h2>Hey, I am Second Page</h2>
<Link to= '/' >Click Me for Back</Link>
</div>
)
}
|
Output:
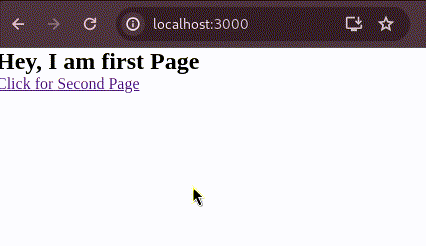
Conclusion:
Now, we know we use ‘Link’ component instead of anchor tag. It is prefered choice in React Application utilizing React Router. It seamlessly integrates with React Router, enabling client-side navigation within single-page applications, preventing full page reloads, and providing additional features for a smoother and more efficient user experience.
Please Login to comment...