How to Convert Blob Data to JSON in JavaScript ?
Last Updated :
23 Apr, 2024
When dealing with Blob data in JavaScript, such as binary data or files, we may need to convert it into JSON format for doing so JavaScript provides us with various methods as listed below.
Using FileReader API
In this approach, we first use the readAsText()
method to read the Blob as text and once the data is loaded we parse the text into JSON using JSON.parse()
.
Example: The below code implements the FileReader API to convert the blob data into JSON format.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content=
"width=device-width, initial-scale=1.0">
<title>
Converting Blob to JSON
</title>
</head>
<body style="text-align: center;">
<h2>
Converting "{\"name\": \"John\",
\"age\": 30}"
<br/>string to Blob and then
formatting it into JSON
</h2>
<h3 id="json"></h3>
<script>
const jsonHeading =
document.getElementById('json');
const blob = new Blob(
["{\"name\": \"John\", \"age\": 30}"],
{ type: "application/json" });
const reader = new FileReader();
reader.onload = function (event) {
const jsonData =
JSON.parse(event.target.result);
jsonHeading.innerHTML =
"Converted JSON Data = { Name: " +
jsonData.name + ", Age: " +
jsonData.age + " }";
};
reader.readAsText(blob);
</script>
</body>
</html>
Output:
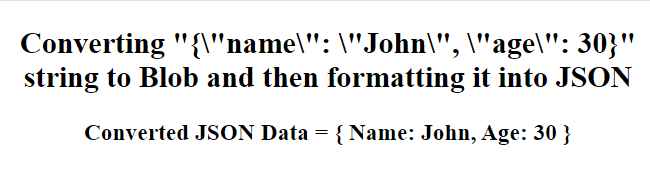
Using TextDecoder API
In this approach, we will use the decode()
method of TextDecoder to decode the Blob data as text and once the data is decoded we parse the text into JSON using JSON.parse().
Example: The below code implements the TextDecoder API to convert blob data into JSON.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content=
"width=device-width, initial-scale=1.0">
<title>
Converting Blob to JSON
</title>
</head>
<body style="text-align: center;">
<h2>
Converting "{\"name\": \"John\",
\"age\": 30}"
<br />string to Blob and then
formatting it into JSON
</h2>
<h3 id="json"></h3>
<script>
const jsonHeading =
document.getElementById('json');
const blob = new Blob(
["{\"name\": \"John\", \"age\": 30}"],
{ type: "application/json" });
const reader = new FileReader();
reader.onload = function (event) {
const textDecoder = new TextDecoder();
const decodedText =
textDecoder.decode(event.target.result);
const jsonData = JSON.parse(decodedText);
jsonHeading.innerHTML =
"Converted JSON Data = { Name: " +
jsonData.name + ", Age: " +
jsonData.age + " }";
};
reader.readAsArrayBuffer(blob);
</script>
</body>
</html>
Output:
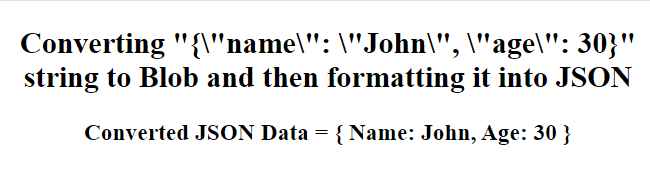
Please Login to comment...