How to pass form variables from one page to other page in PHP ?
Last Updated :
31 Jul, 2021
Form is an HTML element used to collect information from the user in a sequential and organized manner. This information can be sent to the back-end services if required by them, or it can also be stored in a database using DBMS like MySQL. Splitting a form into multiple steps or pages allow better data handling and layering of information. This can be achieved by creating browser sessions. HTML sessions are a collection of variables that can be used to maintain the state of the form attributes while the user switched between the pages of the current domain. Session entries will be deleted as soon as the user closes the browser or leaves the site.
Syntax:
<?php
session_start();
session_register('variable_name');
$_SESSION['variable_name']=variable_value;
?>
Example: This example will illustrate the steps to create a three-paged form using PHP and Browser Sessions. It is in reference to a coaching institute’s registration form. The first page of the form will be asking the user to enter their name, email and mobile number, which will be transferred to another PHP page. Where the information will be stored into session directories.
- Code 1: Start your localhost server like Apache, etc. Complete writing the HTML tags and write the below code inside the BODY section. Save the file with the format ‘form1.php’ in the local directory of your localhost. Open your web browser and type your localhost address followed by ‘\form1.php’.
<form method= "POST" action= "form2.php" >
<pre>Name: <input type= "text"
name= "user_name" >
</pre>
<pre>Email Address: <input type= "text"
name= "user_email_address" >
</pre>
<pre>Mobile Number: <input type= "number"
name= "user_mobile_number" >
</pre>
<input type= "submit" value= "Next" >
</form>
|
- Output: It will open your form like this, asked information will be passed to the PHP page linked with the form (action=”form2.php”) with the use of the POST method. In the next step, submitted information will be stored in the session array.
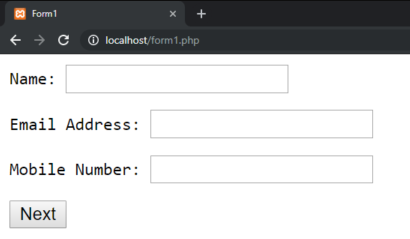
- Code 2: Repeat the process of saving the file as explained above. Use the file name ‘form2.php’. When you will click ‘Next’ on form1.php page. This page will be asking the college/company name, city, state user is in, and the course he/she is applying for.
<?php
session_start();
$_SESSION [ 'name' ] = $_POST [ 'user_name' ];
$_SESSION [ 'email_address' ]
= $_POST [ 'user_email_address' ];
$_SESSION [ 'mobile_number' ]
= $_POST [ 'user_mobile_number' ];
?>
<!-- Form for other details-->
<form method= "POST" action= "form3.php" >
<pre>
Company/College:
<input type= "text" name= "college_name" >
</pre>
<pre>
City:
<input type= "text" name= "city" >
</pre>
<pre>
State:
<input type= "text" name= "state" >
</pre>
<pre>
You're a:
<input type= "radio" name= "profession"
value= "Student" >Student
<input type= "radio" name= "profession"
value= "Working Professional" >
Working Professional
</pre>
<pre>
Course:
<select name= "course" >
<option value= "DSnA" >
Data Structures and Algorithms
</option>
<option value= "Gate_test" >
GATE Mock Test
</option>
<option value= "Mock_interview" >
Mock Interviews
</option>
<option value= "Machine_learning" >
Machine Learning
</option>
</select>
</pre>
<br>
<pre>
<input type= "checkbox"
name= "terms_and_conditions" >
Terms and Conditions
</pre>
<br>
<input type= "submit" value= "Register" >
</form>
|
- Output: It will be redirected you to this page, which will look like this:
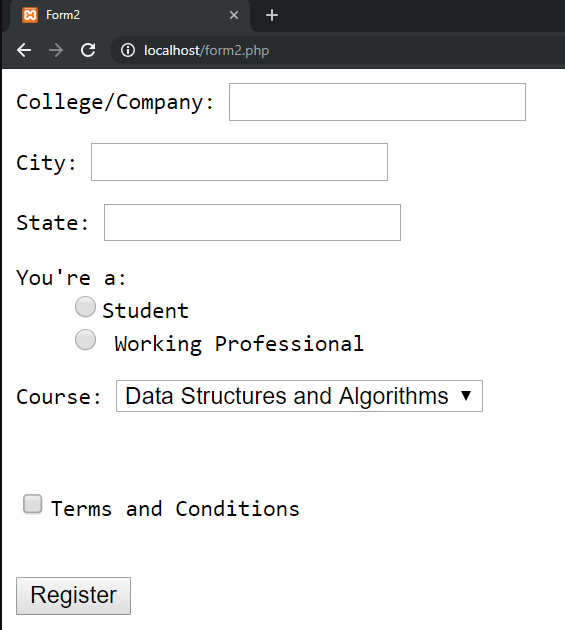
- Code 3: In this step, we will be extracting the information from the session array and storing it on our MySQL Database. Make a third file named ‘form3.php’ and write the following code inside the BODY section, and apply the necessary HTML tags.
<?php
session_start();
$insert_query = 'insert into subscriptions (
name,
email_address,
mobile_number,
college_name,
city,
state,
profession,
course,
terms_and_conditions,
) values (
' . $_SESSION[' name'] . ",
" . $_SESSION['email_address'] . " ,
" . $_SESSION['mobile_number'] . " ,
" . $_POST['college_name']. " ,
" . $_POST['city']. " ,
" . $_POST['state']. " ,
" . $_POST['profession']. " ,
" . $_POST['course']. " ,
" . $_POST['terms_and_conditions']. "
);"
mysql_query( $insert_query );
?>
<pre>Successfully Registered</pre>
|
- Output: On clicking Register on page 2, it will redirect you to this page where your data will be submitted into the database. As a prerequisite, you have to link your page to a MySQL database. For that, you may refer this
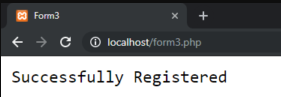
Conclusion: Sessions can be used to keep form data active until either the browser is closed or the site is left. Please note that while writing the final query, we have used data from the $_SESSION array, and also data from the $_POST array, that was posted from the last step of the form.
PHP is a server-side scripting language designed specifically for web development. You can learn PHP from the ground up by following this PHP Tutorial and PHP Examples.
Please Login to comment...