PyQt5 QSpinBox – Removing the arrow buttons
Last Updated :
06 May, 2020
In this article we will see how we can remove the buttons of the spin box, basically there are two buttons in the spin box one for incrementing the value and second for decrementing the value. Below is the representation of how normal spin box looks like vs the spin box with no buttons looks like
In order to do this we will use setButtonSymbols
method.
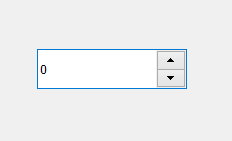
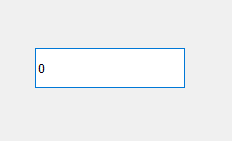
Syntax :
spin_box.setButtonSymbols(QAbstractSpinBox.NoButtons)
or
spin_box.setButtonSymbols(2)
Argument : It takes either QAbstractSpinBox object or integer value
Return : None
Below is the implementation
from PyQt5.QtWidgets import *
from PyQt5 import QtCore, QtGui
from PyQt5.QtGui import *
from PyQt5.QtCore import *
import sys
class Window(QMainWindow):
def __init__( self ):
super ().__init__()
self .setWindowTitle( "Python " )
self .setGeometry( 100 , 100 , 600 , 400 )
self .UiComponents()
self .show()
def UiComponents( self ):
self .spin = QSpinBox( self )
self .spin.setGeometry( 100 , 100 , 150 , 40 )
self .spin.setButtonSymbols(QAbstractSpinBox.NoButtons)
App = QApplication(sys.argv)
window = Window()
sys.exit(App. exec ())
|
Output :
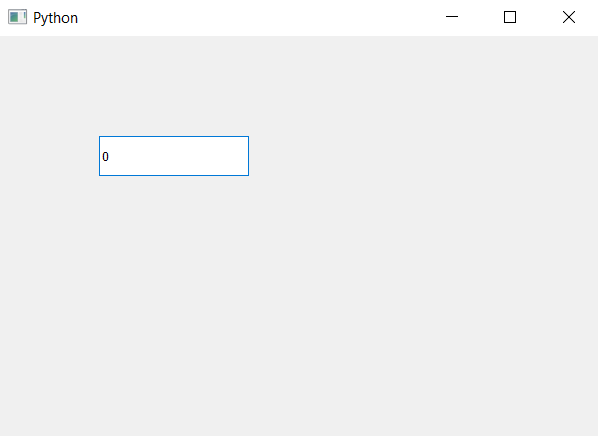
Please Login to comment...