Add Matrix in C
Last Updated :
31 Jul, 2023
Matrices are the collection of numbers arranged in order of rows and columns. In this article, we will learn to write a C program for the addition of two matrices.
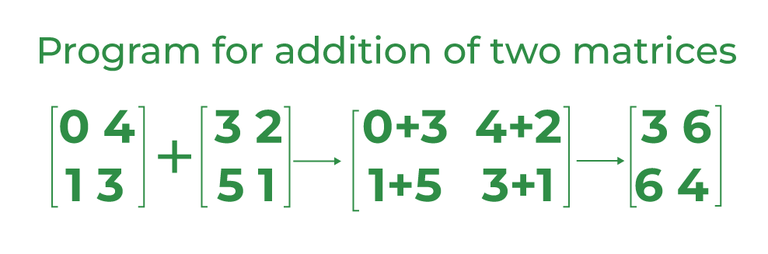
The idea is to use two nested loops to iterate over each element of the matrices. The addition operation is performed by adding the corresponding elements of mat1[] and mat2[] and storing the result in the corresponding position of the resultant matrix.
C Program to Add Two Square Matrices
The below program adds two square matrices of size 4*4, we can change N for different dimensions.
C
#include <stdio.h>
#define N 4
void add( int A[][N], int B[][N], int C[][N])
{
int i, j;
for (i = 0; i < N; i++)
for (j = 0; j < N; j++)
C[i][j] = A[i][j] + B[i][j];
}
void printmatrix( int D[][N])
{
int i, j;
for (i = 0; i < N; i++) {
for (j = 0; j < N; j++)
printf ( "%d " , D[i][j]);
printf ( "\n" );
}
}
int main()
{
int A[N][N] = { { 1, 1, 1, 1 },
{ 2, 2, 2, 2 },
{ 3, 3, 3, 3 },
{ 4, 4, 4, 4 } };
int B[N][N] = { { 1, 1, 1, 1 },
{ 2, 2, 2, 2 },
{ 3, 3, 3, 3 },
{ 4, 4, 4, 4 } };
int C[N][N];
int i, j;
printf ( "Matrix A is \n" );
printmatrix(A);
printf ( "Matrix B is \n" );
printmatrix(B);
add(A, B, C);
printf ( "Result matrix is \n" );
printmatrix(C);
return 0;
}
|
Output
Matrix A is
1 1 1 1
2 2 2 2
3 3 3 3
4 4 4 4
Matrix B is
1 1 1 1
2 2 2 2
3 3 3 3
4 4 4 4
Result matrix is
2 2 2 2
4 4 4 4
6 6 6 6
8 8 8 8
Complexity Analysis
- Time Complexity: O(n2)
- Auxiliary Space: O(n2)
C Program to Add Two Rectangular Matrices
Below is the C program to add two rectangular matrices.
C
#include <stdio.h>
#define M 4
#define N 3
void add( int A[M][N], int B[M][N], int C[M][N])
{
int i, j;
for (i = 0; i < M; i++)
for (j = 0; j < N; j++)
C[i][j] = A[i][j] + B[i][j];
}
void printmatrix( int D[M][N])
{
int i, j;
for (i = 0; i < M; i++) {
for (j = 0; j < N; j++)
printf ( "%d " , D[i][j]);
printf ( "\n" );
}
}
int main()
{
int A[M][N] = {
{ 1, 1, 1 }, { 2, 2, 2 }, { 3, 3, 3 }, { 4, 4, 4 }
};
int B[M][N] = {
{ 2, 1, 1 }, { 1, 2, 2 }, { 2, 3, 3 }, { 3, 4, 4 }
};
printf ( "Matrix A is \n" );
printmatrix(A);
printf ( "Matrix B is \n" );
printmatrix(B);
int C[M][N];
int i, j;
add(A, B, C);
printf ( "Result matrix is \n" );
printmatrix(C);
return 0;
}
|
Output
Matrix A is
1 1 1
2 2 2
3 3 3
4 4 4
Matrix B is
2 1 1
1 2 2
2 3 3
3 4 4
Result matrix is
3 2 2
3 4 4
5 6 6
7 8 8
Complexity Analysis
- Time Complexity: O(m*n)
- Auxiliary Space: O(m*n)
Refer to the complete article Program for addition of two matrices for more details!
Related Articles
Share your thoughts in the comments
Please Login to comment...