8086 program to find sum of Even numbers in a given series
Last Updated :
28 May, 2018
Problem – Write a program in 8086 microprocessor to find out the sum of series of even numbers, where numbers are stored from starting offset 500 and store the result at offset 600.
Example –
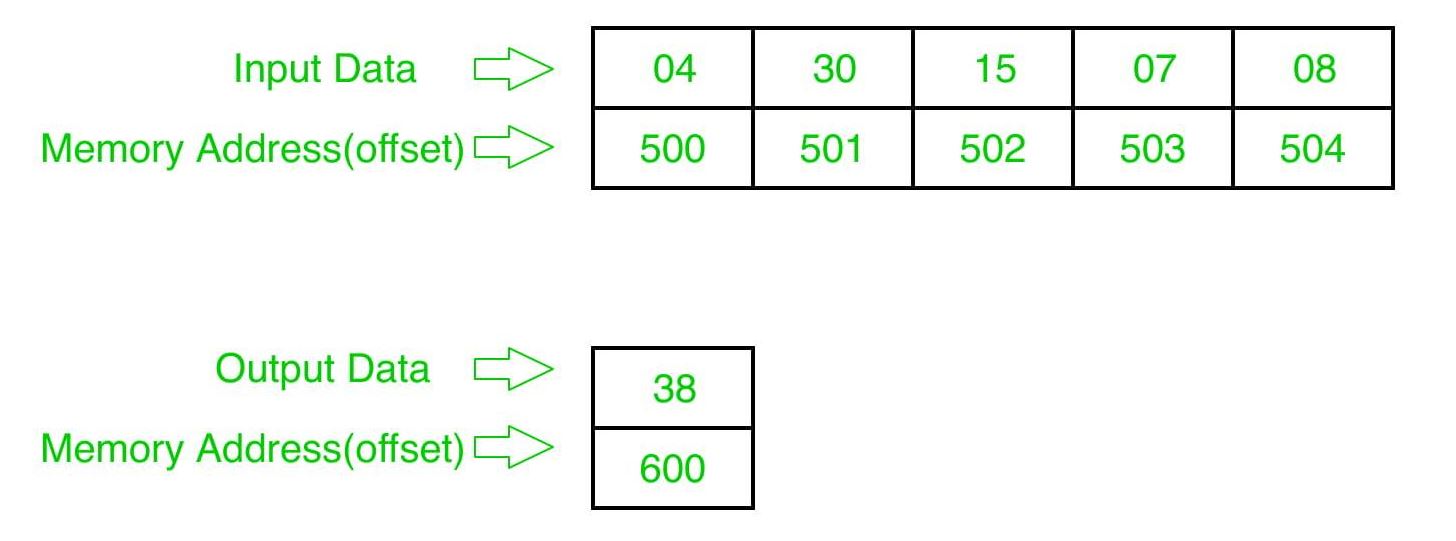
Algorithm –
- Assign 500 to SI
- Load data from offset SI to register CL (count) and assign 00 to register CH inc. SI by 1
- Load data from offset SI and apply TEST with 01, if result is non zero jump to step 5
- Add the offset data with register AL
- Increase offset by 1
- LOOP to step 3
- Store the result (content of register AL) to offset 600
- Stop
Program –
MEMORY ADDRESS |
MNEMONICS |
COMMENT |
400 |
MOV SI, 500 |
SI<-500 |
403 |
MOV CL, [SI] |
CL<-[SI] |
405 |
INC SI |
SI<-SI+1 |
406 |
MOV CH, 00 |
CH<-00 |
408 |
MOV AL, 00 |
AL<-00 |
40A |
MOV BL, [SI] |
BL<-[SI] |
40C |
TEST BL, 01 |
BL AND 01 |
40F |
JNZ 413 |
JUMP IF NOT ZERO |
411 |
ADD AL, BL |
AL<-AL+BL |
413 |
INC SI |
SI<-SI+1 |
414 |
LOOP 40A |
JUMP TO 40A IF CX NOT ZERO |
416 |
MOV [600], AL |
AL->[600] |
41A |
HLT |
END |
Explanation –
- MOV SI, 500: assign 500 to SI
- MOV CL, [SI]: load data from offset SI to register CL
- INC SI: increase value of SI by 1
- MOV CH, 00: assign 00 to register CH
- MOV AL, 00: assign 00 to register AL
- MOV BL, [SI]: load data from offset SI to register BL
- TEST BL, 01: AND register BL with 01
- JNZ 413: jump to address 413 if not zero
- ADD AL, BL: add contents of register AL and BL
- INC SI: increase value of SI by 1
- LOOP 40A: jump to 40A if CX not zero and CX=CX-1
- MOV [600], AL: store the value of register AL to offset 600
- HLT: end.
Refer for – 8086 program to find sum of odd numbers in a given series
Share your thoughts in the comments
Please Login to comment...