Adjacency Matrix
Last Updated :
29 Apr, 2024
Adjacency Matrix is a square matrix used to represent a finite graph. The elements of the matrix indicate whether pairs of vertices are adjacent or not in the graph. An adjacency matrix is a simple and straightforward way to represent graphs and is particularly useful for dense graphs.
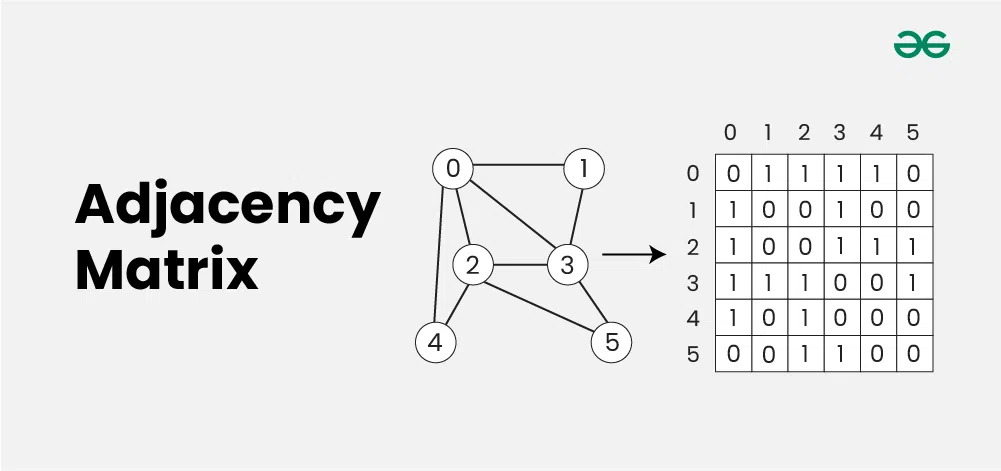
What is Adjacency Matrix?
Adjacency Matrix is a square matrix used to represent a finite graph by storing the relationships between the nodes in their respective cells. For a graph with V vertices, the adjacency matrix A is an V X V matrix where:
- A[i][j] = 1, if there is an edge between vertex i and vertex j
- A[i][j] = 0, if there is no edge between vertex i and vertex j
1. Adjacency Matrix for Undirected and Unweighted graph:
Consider an Undirected and Unweighted graph G with 4 vertices and 3 edges. For the graph G, the adjacency matrix would look like:
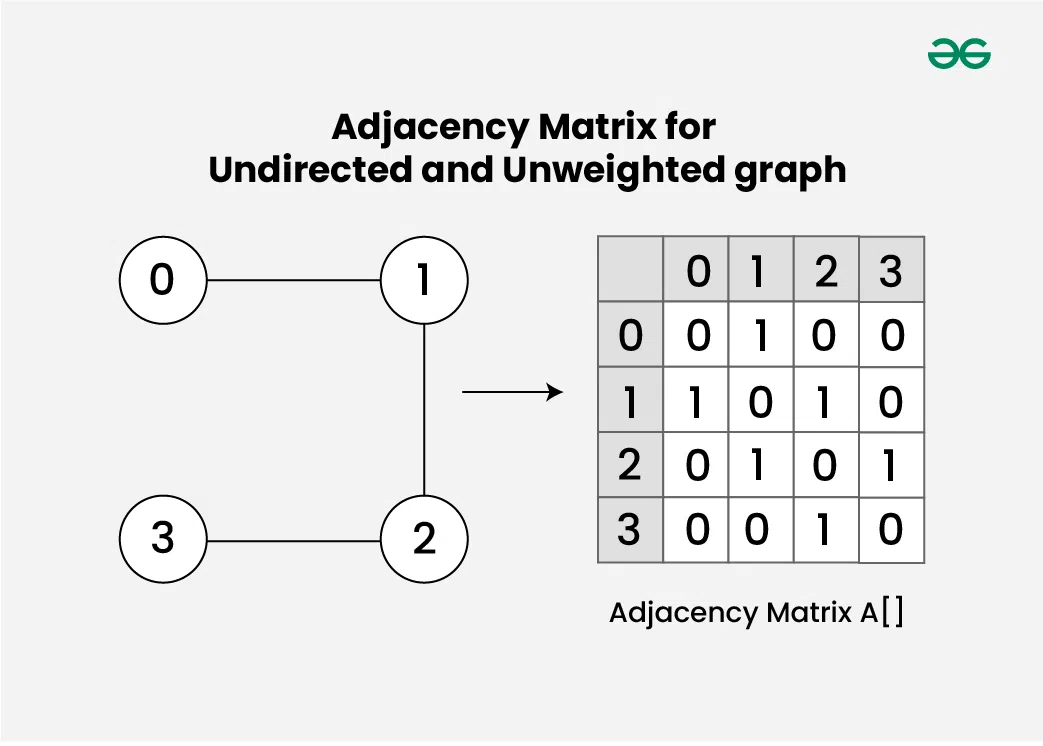
Here’s how to interpret the matrix:
- A[0][1] ​= 1, there is an edge between vertex 0 and vertex 1.
- A[1][0] ​= 1, there is an edge between vertex 1 and vertex 0.
- A[1][2] ​= 1, there is an edge between vertex 1 and vertex 2.
- A[2][1] ​= 1, there is an edge between vertex 2 and vertex 1.
- A[2][3] = 1, there is an edge between vertex 2 and vertex 3.
- A[3][2] = 1, there is an edge between vertex 3 and vertex 2.
- A[i][i] = 0, as there are no self loops on the graph.
- All other entries with a value of 0 indicate no edge between the corresponding vertices.
2. Adjacency Matrix for Undirected and Weighted graph:
Consider an Undirected and Weighted graph G with 5 vertices and 5 edges. For the graph G, the adjacency matrix would look like:
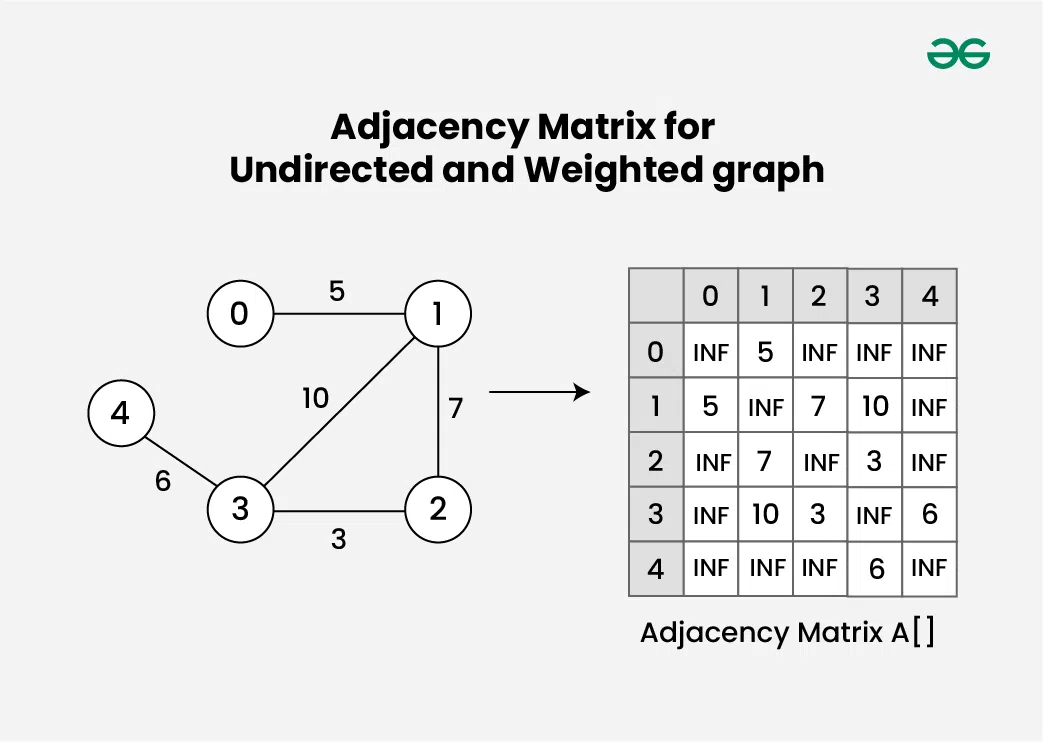
Here’s how to interpret the matrix:
- A[0][1] ​= 5, there is an edge between vertex 0 and vertex 1 having weight = 5.
- A[1][0] ​= 5, there is an edge between vertex 1 and vertex 0 having weight = 5.
- A[1][2] ​= 7, there is an edge between vertex 1 and vertex 2 having weight = 7.
- A[2][1] ​= 7, there is an edge between vertex 2 and vertex 1 having weight = 7.
- A[2][3] ​= 3, there is an edge between vertex 2 and vertex 3 having weight = 3.
- A[3][2] ​= 3, there is an edge between vertex 3 and vertex 2 having weight = 3.
- A[1][3] = 10, there is an edge between vertex 1 and vertex 3 having weight = 10.
- A[3][1] = 10, there is an edge between vertex 3 and vertex 1 having weight = 10.
- A[3][4] = 6, there is an edge between vertex 3 and vertex 4 having weight = 6.
- A[4][3] = 6, there is an edge between vertex 4 and vertex 3 having weight = 6.
- A[i][i] = INF, as there are no self loops on the graph. We can also initialize A[i][i] = 0 as starting from any node i, we don’t need to traverse any edge to reach node i.
- All other entries with a value of INF indicate no edge between the corresponding vertices.
3. Adjacency Matrix for Directed and Unweighted graph:
Consider an Directed and Unweighted graph G with 4 vertices and 4 edges. For the graph G, the adjacency matrix would look like:
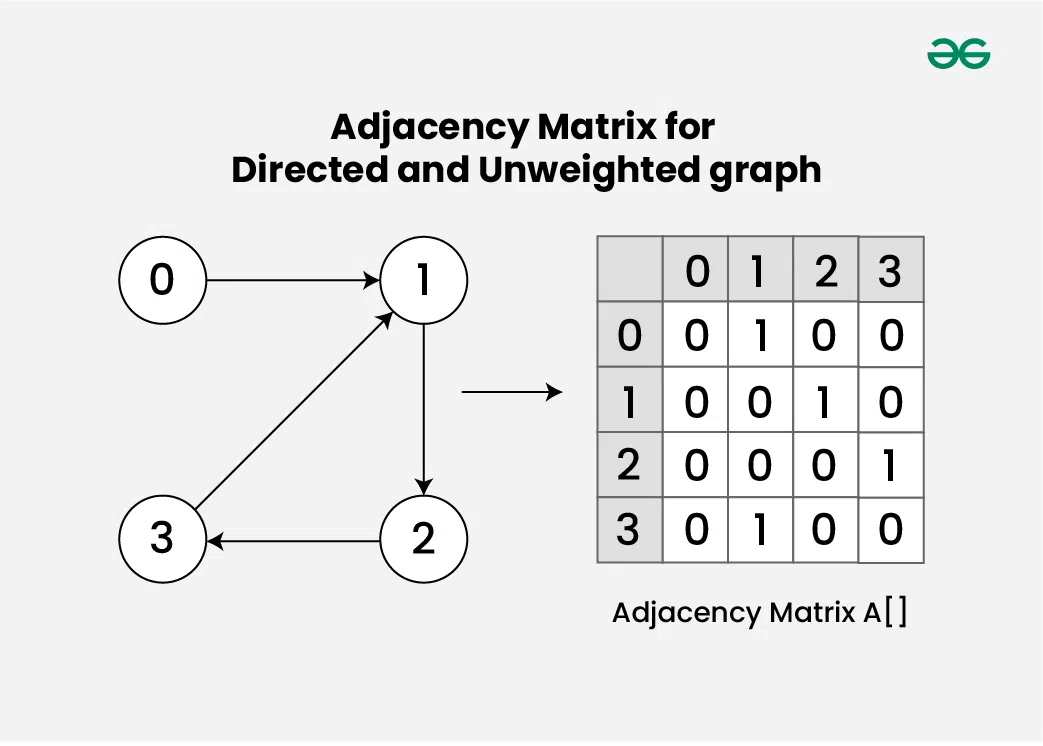
Here’s how to interpret the matrix:
- A[0][1] ​= 1, there is an edge between vertex 0 and vertex 1.
- A[1][2] ​= 1, there is an edge between vertex 1 and vertex 2.
- A[2][3] = 1, there is an edge between vertex 2 and vertex 3.
- A[3][1] = 1, there is an edge between vertex 3 and vertex 1.
- A[i][i] = 0, as there are no self loops on the graph.
- All other entries with a value of 0 indicate no edge between the corresponding vertices.
4. Adjacency Matrix for Directed and Weighted graph:
Consider an Directed and Weighted graph G with 5 vertices and 6 edges. For the graph G, the adjacency matrix would look like:
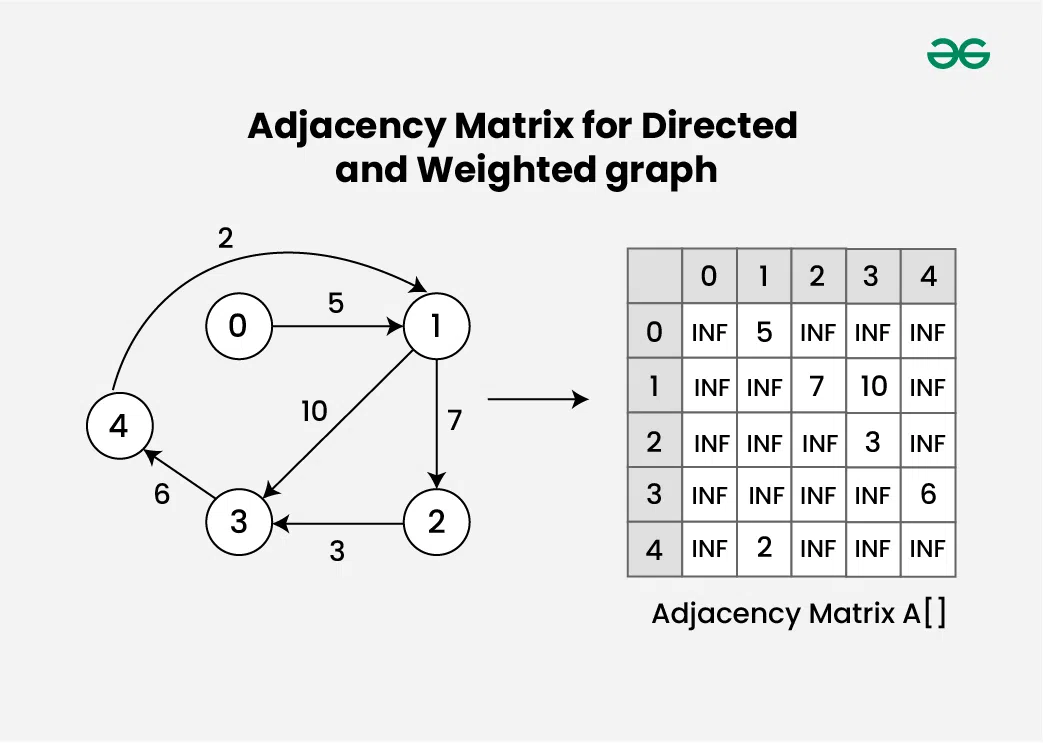
Here’s how to interpret the matrix:
- A[0][1] ​= 1, there is an edge between vertex 0 and vertex 1.
- A[1][2] ​= 1, there is an edge between vertex 1 and vertex 2.
- A[2][3] = 1, there is an edge between vertex 2 and vertex 3.
- A[3][1] = 1, there is an edge between vertex 3 and vertex 1.
- A[i][i] = 0, as there are no self loops on the graph.
- All other entries with a value of 0 indicate no edge between the corresponding vertices.
Implementation of Adjacency Matrix:
Below is a program to create an adjacency matrix for an undirected graph:
C++
#include <iostream>
#include <vector>
using namespace std;
vector<vector<int> >
createAdjacencyMatrix(vector<vector<int> >& graph,
int numVertices)
{
// Initialize the adjacency matrix with zeros
vector<vector<int> > adjMatrix(
numVertices, vector<int>(numVertices, 0));
// Fill the adjacency matrix based on the edges in the
// graph
for (int i = 0; i < numVertices; ++i) {
for (int j = 0; j < numVertices; ++j) {
if (graph[i][j] == 1) {
adjMatrix[i][j] = 1;
adjMatrix[j][i]
= 1; // For undirected graph, set
// symmetric entries
}
}
}
return adjMatrix;
}
int main()
{
// Example graph represented as an adjacency list
// The indices represent the vertices, and the values
// are lists of neighboring vertices
vector<vector<int> > graph = { { 0, 1, 0, 0 },
{ 1, 0, 1, 0 },
{ 0, 1, 0, 1 },
{ 0, 0, 1, 0 } };
int numVertices = graph.size();
// Create the adjacency matrix
vector<vector<int> > adjMatrix
= createAdjacencyMatrix(graph, numVertices);
// Print the adjacency matrix
for (int i = 0; i < numVertices; ++i) {
for (int j = 0; j < numVertices; ++j) {
cout << adjMatrix[i][j] << " ";
}
cout << endl;
}
return 0;
}
Java
import java.util.ArrayList;
import java.util.Arrays;
public class AdjacencyMatrix {
public static int[][] createAdjacencyMatrix(
ArrayList<ArrayList<Integer> > graph,
int numVertices)
{
// Initialize the adjacency matrix with zeros
int[][] adjMatrix
= new int[numVertices][numVertices];
// Fill the adjacency matrix based on the edges in
// the graph
for (int i = 0; i < numVertices; ++i) {
for (int j = 0; j < numVertices; ++j) {
if (graph.get(i).get(j) == 1) {
adjMatrix[i][j] = 1;
adjMatrix[j][i]
= 1; // For undirected graph, set
// symmetric entries
}
}
}
return adjMatrix;
}
public static void main(String[] args)
{
// Example graph represented as an adjacency list
// The indices represent the vertices, and the
// values are lists of neighboring vertices
ArrayList<ArrayList<Integer> > graph
= new ArrayList<>();
graph.add(
new ArrayList<>(Arrays.asList(0, 1, 0, 0)));
graph.add(
new ArrayList<>(Arrays.asList(1, 0, 1, 0)));
graph.add(
new ArrayList<>(Arrays.asList(0, 1, 0, 1)));
graph.add(
new ArrayList<>(Arrays.asList(0, 0, 1, 0)));
int numVertices = graph.size();
// Create the adjacency matrix
int[][] adjMatrix
= createAdjacencyMatrix(graph, numVertices);
// Print the adjacency matrix
for (int i = 0; i < numVertices; ++i) {
for (int j = 0; j < numVertices; ++j) {
System.out.print(adjMatrix[i][j] + " ");
}
System.out.println();
}
}
}
// This code is contributed by shivamgupta310570
Python3
def create_adjacency_matrix(graph):
# Get the number of vertices in the graph
num_vertices = len(graph)
# Initialize the adjacency matrix with zeros
adj_matrix = [[0] * num_vertices for _ in range(num_vertices)]
# Fill the adjacency matrix based on the edges in the graph
for i in range(num_vertices):
for j in range(num_vertices):
if graph[i][j] == 1:
adj_matrix[i][j] = 1
# For undirected graph, set symmetric entries
adj_matrix[j][i] = 1
return adj_matrix
# Example graph represented as an adjacency list
# The indices represent the vertices, and the values are lists of neighboring vertices
graph = [
[0, 1, 0, 0],
[1, 0, 1, 0],
[0, 1, 0, 1],
[0, 0, 1, 0]
]
# Create the adjacency matrix
adj_matrix = create_adjacency_matrix(graph)
# Print the adjacency matrix
for row in adj_matrix:
print(' '.join(map(str, row)))
# This code is contributed by shivamgupta0987654321
JavaScript
function createAdjacencyMatrix(graph) {
const numVertices = graph.length;
// Initialize the adjacency matrix with zeros
const adjMatrix = Array.from(Array(numVertices), () => Array(numVertices).fill(0));
// Fill the adjacency matrix based on the edges in the graph
for (let i = 0; i < numVertices; ++i) {
for (let j = 0; j < numVertices; ++j) {
if (graph[i][j] === 1) {
adjMatrix[i][j] = 1;
adjMatrix[j][i] = 1; // For undirected graph, set symmetric entries
}
}
}
return adjMatrix;
}
// Example graph represented as an adjacency list
// The indices represent the vertices, and the values are lists of neighboring vertices
const graph = [
[0, 1, 0, 0],
[1, 0, 1, 0],
[0, 1, 0, 1],
[0, 0, 1, 0]
];
// Create the adjacency matrix
const adjMatrix = createAdjacencyMatrix(graph);
// Print the adjacency matrix
for (let i = 0; i < adjMatrix.length; ++i) {
console.log(adjMatrix[i].join(' '));
}
Output0 1 0 0
1 0 1 0
0 1 0 1
0 0 1 0
Properties of Adjacency Matrix:
- Diagonal Entries: The diagonal entries A[i][i] are usually set to 0, assuming the graph has no self-loops.
- Undirected Graphs: For undirected graphs, the adjacency matrix is symmetric. This means A[i][j] ​= A[j][i]​ for all i and j.
- Weighted Graphs: The adjacency matrix can also be used to represent weighted graphs by storing the weight of the edge instead of 1 or 0.
Applications of Adjacency Matrix:
- Graph Representation: The adjacency matrix is one of the most common ways to represent a graph computationally.
- Connectivity: By examining the entries of the adjacency matrix, one can determine whether the graph is connected or not. If the graph is undirected, it is connected if and only if the corresponding adjacency matrix is irreducible (i.e., there is a path between every pair of vertices). In directed graphs, connectivity can be analyzed using concepts like strongly connected components.
- Degree of Vertices: The degree of a vertex in a graph is the number of edges incident to it. In an undirected graph, the degree of a vertex can be calculated by summing the entries in the corresponding row (or column) of the adjacency matrix. In a directed graph, the in-degree and out-degree of a vertex can be similarly determined.
Advantages of Adjacency Matrix:
- Simple: Simple and Easy to implement.
- Space Efficient for Dense Graphs: Space efficient when the graph is dense as it requires V * V space to represent the entire graph.
- Faster access to Edges: Adjacency Matrix allows constant look up to check whether there exists an edge between a pair of vertices.
Disadvantages of Adjacency Matrix:
- Space inefficient for Sparse Graphs: Takes up O(V* V) space even if the graph is sparse.
- Costly Insertions and Deletions: Adding or deleting a vertex can be costly as it requires resizing the matrix.
- Slow Traversals: Graph traversals like DFS, BFS takes O(V * V) time to visit all the vertices whereas Adjacency List takes only O(V + E).
Share your thoughts in the comments
Please Login to comment...