Angular Material List
Last Updated :
22 Nov, 2022
Angular Material is a UI component library developed by Google so that Angular developers can develop modern applications in a structured and responsive way. By making use of this library, we can greatly increase the user experience of an end-user thereby gaining popularity for our application. This library contains modern ready-to-use elements which can be directly used with minimum or no extra code.
The <mat-list>is a directive in Angular Material that acts as a container component, which wraps the list of <mat-list-item>in it. Angular Material provides different types of list formats, which are described below:
- Simple lists: A simple list is used to display list items, that can be created using the <mat-list> directive, along with using the <mat-list-item> directive to create a list item.
- Navigation lists: A navigation list is used to navigate to another page when a list item is clicked. In order to create a navigation list, we use the <mat-nav-list> directive.
- Action lists: An action list is used when we want to perform some action while we click on the list item like save. In order to create an action list, we use the <mat-action-list> directive and list items are enclosed within the <button> element.
- Selection lists: A selection list is used to select the list items. To create a selection list, we use <mat-selection-list> & <mat-list-option> directives.
- Multi-line lists: A multi-line list is used when we want to display multiple lines within the same list of items. To create a multi-line list, the matlin attribute is used along with HTML elements like heading tags(<h1>) and paragraph tags(<p>).
- Lists with icons: We can display icons along with list items. We can create a list with icons<mat-icon> directive, where the matListIcon attribute can be used.
- Lists with avatars: We can display avatars along with list items. We can create a list with avatars <img>tag where the matListAvatar attribute can be used.
- Dense lists: We can display list items in dense mode. To create dense lists, the dense attribute with <mat-list> directive can be used.
- Lists with multiple sections: We can display a list with multiple sections. To create a list with multiple sections, a sub-header can be added to a list by a heading tag with a matSubheader attribute. We can also use <mat-divider> to add a divider.
Syntax:
<mat-list>
<mat-list-item>Sample list item</mat-list-item>
...
</mat-list>
Installation Syntax: The basic pre-requisite is that we must have Angular CLI installed on the system in order to add and configure the Angular material library. The following command is executed on the Angular CLI to install the angular material library:
ng add @angular/material
Make sure the path should be opened in the terminal before executing the above command.
Please refer to the Adding Angular Material Component to Angular Application article for the detailed installation procedure.
Adding List Component: To use the List Component, we need to import it into the app.module.ts file:
import { MatListModule } from '@angular/material/list';
To use the list component in our code we have to import MatListModule into the imports array.
Project Structure: After successful installation, the project structure will look like the following image:
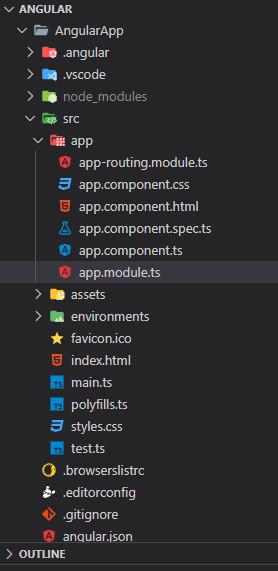
Project Structure
Example 1: This example describes the basic usage of the Angular Material List by implementing the Navigation List.
Javascript
import { NgModule } from "@angular/core" ;
import { BrowserModule } from "@angular/platform-browser" ;
import { BrowserAnimationsModule }
from "@angular/platform-browser/animations" ;
import { AppComponent } from "./app.component" ;
import { MatListModule } from '@angular/material/list' ;
import { FormsModule } from "@angular/forms" ;
@NgModule({
imports: [
BrowserModule,
FormsModule,
MatListModule,
BrowserAnimationsModule,
],
declarations: [AppComponent],
bootstrap: [AppComponent],
})
export class AppModule { }
|
HTML
< div >
< h1 >GeeksforGeeks</ h1 >
< h3 >Angular Material Navigation List</ h3 >
< div >
< mat-nav-list >
React
</ a >
Angular
</ a >
</ mat-nav-list >
</ div >
</ div >
|
Javascript
import { Component } from "@angular/core" ;
@Component({
selector: "my-app" ,
templateUrl: "./app.component.html" ,
styleUrls: [ "./app.component.css" ],
})
export class AppComponent {
title = "AngularApp" ;
}
|
Output:
Example 2: This example describes the basic usage of the Simple list, Action List & Selection list in Angular Material.
Javascript
import { NgModule } from "@angular/core" ;
import { BrowserModule } from "@angular/platform-browser" ;
import { BrowserAnimationsModule }
from "@angular/platform-browser/animations" ;
import { AppComponent } from "./app.component" ;
import { MatListModule } from '@angular/material/list' ;
import { FormsModule } from "@angular/forms" ;
@NgModule({
imports: [
BrowserModule,
FormsModule,
MatListModule,
BrowserAnimationsModule,
],
declarations: [AppComponent],
bootstrap: [AppComponent],
})
export class AppModule { }
|
HTML
< div >
< div style = "text-align: center" >
< h1 style = "color: green" >
GeeksforGeeks</ h1 >
< h3 >Angular Material List</ h3 >
</ div >
< h5 >Simple List:</ h5 >
< mat-list >
< mat-list-item > Angular </ mat-list-item >
< mat-list-item > Vue </ mat-list-item >
< mat-list-item > React </ mat-list-item >
</ mat-list >
< h5 > Action List</ h5 >
< mat-action-list >
< button mat-list-item (click)="save()">
Save
</ button >
< button mat-list-item (click)="undo()">
Undo
</ button >
</ mat-action-list >
< h5 > Selection List</ h5 >
< mat-selection-list #css>
< mat-list-option * ngFor = "let type of typesOfCSS" >
{{type}}
</ mat-list-option >
</ mat-selection-list >
< br >
< p >
Options selected: {{css.selectedOptions.selected.length}}
</ p >
</ div >
|
Javascript
import { Component } from '@angular/core' ;
@Component({
selector: 'my-app' ,
templateUrl: './app.component.html' ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
title = 'AngularApp' ;
typesOfCSS: string[] = [ 'CSS' , 'SASS' , 'SCSS' ,
'Bootstrap' , 'Tailwind CSS' ];
save() {
alert( 'Saved!' );
}
undo() {
alert( 'Undo done!' );
}
}
|
Output:
Reference: https://material.angular.io/components/list/overview
Share your thoughts in the comments
Please Login to comment...