goto statement in R Programming
Last Updated :
26 May, 2020
Goto statement in a general programming sense is a command that takes the code to the specified line or block of code provided to it. This is helpful when the need is to jump from one programming section to the other without the use of functions and without creating an abnormal shift.
Unfortunately, R doesn’t support goto but its algorithm can be easily converted to depict its application. By using following methods this can be carried out more smoothly:
- Use of if and else
- Using break, next and return
Flowchart
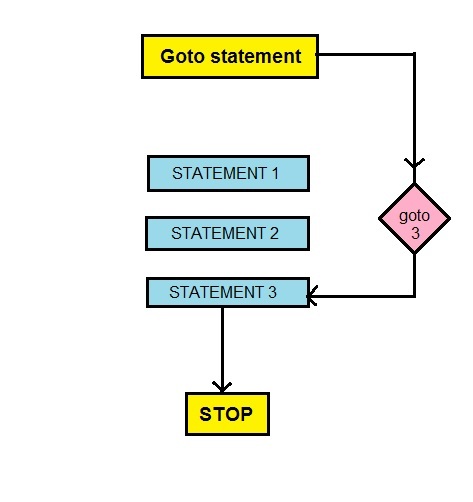
- Goto encountered
- Jump to the specified line number/ name of the code block
- Execute code
Example 1: Program to check for even and odd numbers
a < - 4
if ((a % % 2 ) = = 0 )
{
print ( "even" )
}
else
{
print ( "odd" )
}
|
Output:
[1] "even"
Explanation:
- With goto:
- Two blocks named EVEN and ODD
- Evaluate for a
- if even, goto block named EVEN
- if odd, goto block named ODD
- Without goto:
- Evaluate for a
- if even, run the statement within if block
- if odd, run the statement within else block
Example 2: Program to check for prime numbers
a < - 16
b < - a / 2
flag < - 0
i < - 2
repeat
{
if ((a % % i) = = 0 )
{
flag < - 1
break
}
}
if (flag = = 1 )
{
print ( "composite" )
}
else
{
print ( "prime" )
}
|
Output:
[1] "composite"
Explanation:
- With goto :
- This doesn’t require flag and if statement to check flag.
- Evaluate for a
- If a factor is found take the control to the line number that has the print statement – print(“composite).
- If not take it to the line number that has statement – print(“prime”)
- Without goto:
- Evaluate for a
- If factor found, change flag
- when the loop is complete check flag
- Print accordingly
Note: Since R doesn’t have the concept of the goto statement, the above examples were made using simple if-else and break statements.
Share your thoughts in the comments
Please Login to comment...