Java Multithreading Program with Example
Last Updated :
10 Jan, 2023
Multithreading is a concept in which our program can do multiple tasks in a single unit of time. Thread is the execution unit of any process. Every process must have one thread and that thread name is the main thread. In this article. We will create a Java program that will do writing on file until the user gives input on the terminal. Here, We need two threads. One thread does writing on file and another waits for user input on the terminal.
Java Program and Explanation
Here, One thread is doing writing on file, and the other thread will wait for user input on the terminal. Main class is executed on the parent thread and also it creates a child thread that will do writing on file.
Tasks of Parent thread:
- Creates child thread which writes on file.
- Waits for user input on the terminal.
Tasks of Child thread:
- Do writing on text file until the user gives input on the terminal.
Main.java
Java
import java.lang.*;
import java.util.Scanner;
public class Main {
public static boolean stop = false ;
public static void main(String args[])
{
WriteOnFile newFile = new WriteOnFile(
"Anjali loves GeeksforGeeks." , "File.txt" );
newFile.start();
Scanner sc = new Scanner(System.in);
System.out.print( "Enter Something : " );
sc.nextLine();
Main.stop = true ;
return ;
}
}
|
Explanation of the above code:
- It creates an object of WriteOnFile Class which extends the Thread class.
- After this, it invokes the start() method which executes WriteOnFile run() method on a different thread i.e. child thread.
- After this, It waits for user input on the terminal.
WriteOnFile.java
Java
import java.io.File;
import java.io.FileWriter;
import java.lang.*;
import java.util.Scanner;
class WriteOnFile extends Thread {
private String mssg, fileName;
private String readFile()
{
String currText = "" ;
try {
File myFile = new File( this .fileName);
Scanner myReader = new Scanner(myFile);
while (myReader.hasNextLine()) {
currText += myReader.nextLine();
currText += "\n" ;
}
}
catch (Exception err) {
}
return currText;
}
private void write()
{
while (Main.stop == false ) {
try {
String currText = readFile();
FileWriter myWriter
= new FileWriter(fileName);
myWriter.write(currText + this .mssg);
myWriter.close();
Thread.sleep( 1500 );
}
catch (Exception err) {
}
}
return ;
}
public WriteOnFile(String mssg, String fileName)
{
this .mssg = mssg;
this .fileName = fileName;
}
public void run()
{
this .write();
}
}
|
Explanation of the above code:
- We inherit our class WriteOnFile to Thread class which helps us to make our program multithreaded.
- It’s run() method is invoked internally whenever we invoke the start() method of Main.java.
- The run() method will do writing on the file until the start variable value is true and its value is false until the user has not entered any input on the terminal.
Output:
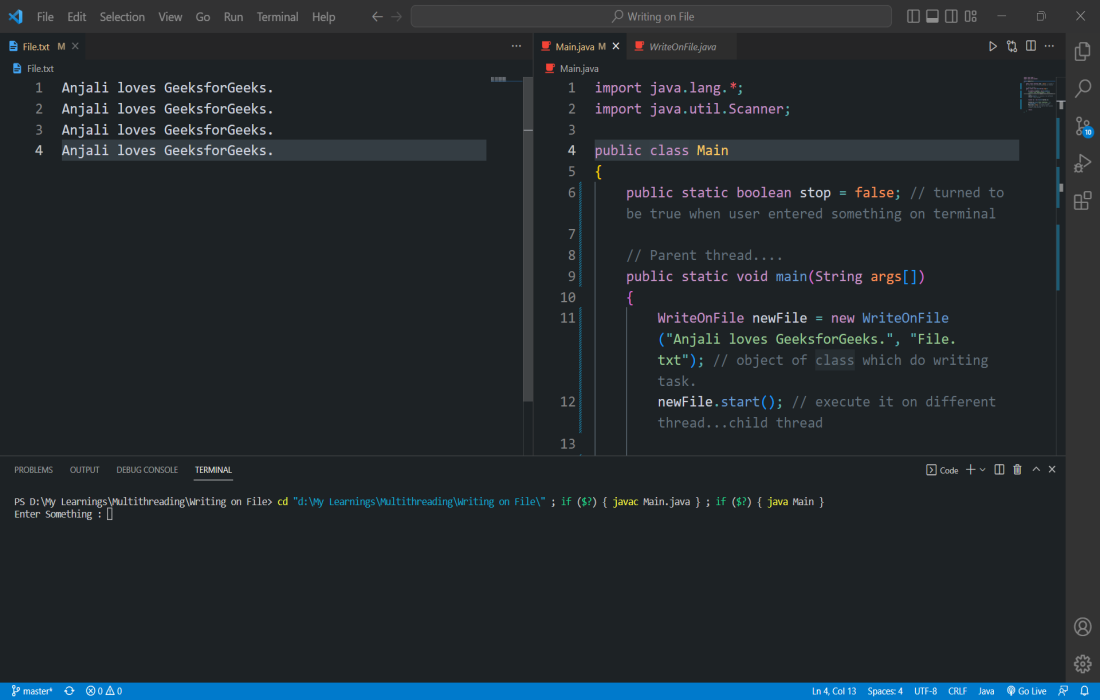
The output of the above demonstration – As you can see main thread waits for input in the terminal and another thread is writing on File.txt
Note: Give the proper path to the text file on which you want to write. Here our file presents in the same folder so we only gave the file name.
Share your thoughts in the comments
Please Login to comment...