JDBC New Features
Last Updated :
09 Apr, 2024
JDBC stands for Java Database Connectivity. It is a crucial technology for Java developers and it facilitates the interaction between the Java applications and the databases. Now, JDBC 4.0 has evolved and introduced new features to improve performance, developer productivity, and security. JDBC has the subsequent updates that have brought about incremental improvements and enhancements. JDBC RowSet provided the disconnected set of rows from the database. JDBC 4.2 introduced the asynchronous database access API. It allowed the developers to execute the database operations asynchronously.
JDBC 4.0 New Features
- Automatic driver loading: JDBC 4.0 introduced automatic driver loading, which removed the need to explicitly load a JDBC driver with Class.forName(). The drivers are automatically installed when the application requests a connection to the database.
- Enhanced exception handling: Advanced exception handling mechanisms provide more detailed and informative error messages, and help developers handle database connection issues more efficiently
- Simplified connection management: JDBC 4.0 simplifies connection management through connection grouping and other enhancements, reducing the cost of establishing and closing connections to the database
- Java SE 6 Integration: Integration of JDBC 4.0 and Java SE 6, with features introduced in Java 6 such as add-on efforts for better resource utilization and improved performance
- Improved Performance: JDBC 4.0 includes enhancements aimed at improving performance, such as reducing latency in executing database queries and optimizing resource utilization
- Enhanced Security Features: JDBC 4.0 introduces enhanced security features, including stronger encryption algorithms and better protection against SQL injection attacks, to ensure data transmission between the application and the database is accurate and confidential
- Support for SQL XML: JDBC 4.0 provides built-in support for processing XML data types, enables seamless integration of XML data with relational databases, and enables flexible and efficient data generation
Prerequisites:
The following are the prerequisites to utilize the latest features of JDBC in your Java applications:
- Java Version Compatibility.
- Driver Compatibility
- Database Compatibility
- Configuration and Setup
- Understanding of JDBC Basics
Note: If you met above prerequisites, developers can effectively use the latest features of JDBC in their Java applications.
Step-by-step Implementation to Utilize New JDBC Features in Java application
Below are the steps to utilize the new JDBC 4.0 features by setting up a Java project.
Step 1: Create table in the Database
Create a table in database and name it as “employees” and column names as “name” ,”age” and “department”. Insert the rows into the table.
Here is the example for “employees” table:
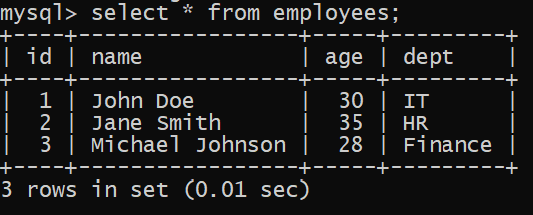
Step 2: Create Java Project in Eclipse
Open Eclipse IDE and create a Java project, name it as which you want as project name.
Step 3: Add MYSQL JDBC Driver to the project
- First download the MYSQL JDBC driver (jar file) from the MYSQL website.
- Open Eclipse, right click on the name of the project in the Package Explorer.
- After that select the Build Path and then Configure Build Path.
- There is Libraries tab and then click Add External JARs and then select the downloaded MYSQL JDBC driver JAR file.
- After that click Apply and then Apply and close button.
Here is the path for MYSQL JDBC driver jar file:
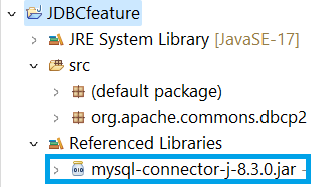
Step 4: Create a Java class in the project
Create a class in the src folder in the java project, and name it as “DataSourceFactory“.
Here is the path for java class file:
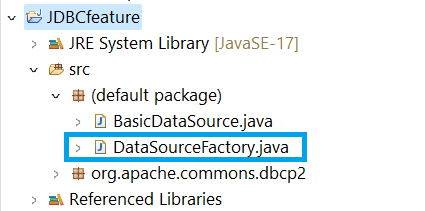
Step 5: Implement the code
Open the java class file and write the below code to retrieve the data from the database.
Java
import javax.sql.DataSource;
import java.io.PrintWriter;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.SQLFeatureNotSupportedException;
import java.util.logging.Logger;
public class DataSourceFactory implements DataSource {
// JDBC connection parameters
private static final String URL = "jdbc:mysql://localhost:3307/work";
private static final String USER = "root";
private static final String PASSWORD = " ";
// method to get connection with the database
@Override
public Connection getConnection() throws SQLException {
// Establish connection using URL, username, and password
return DriverManager.getConnection(URL, USER, PASSWORD);
}
// Method to get a connection to the database with provided username and password
@Override
public Connection getConnection(String username, String password) throws SQLException
{
// Establish connection using URL, provided username, and password
return DriverManager.getConnection(URL, username, password);
}
// Methods below are not implemented for this example
@Override
public Logger getParentLogger() throws SQLFeatureNotSupportedException
{
return null;
}
@Override
public <T> T unwrap(Class<T> iface) throws SQLException
{
return null;
}
@Override
public boolean isWrapperFor(Class<?> iface) throws SQLException
{
return false;
}
@Override
public PrintWriter getLogWriter() throws SQLException
{
return null;
}
@Override
public void setLogWriter(PrintWriter out) throws SQLException
{
}
@Override
public void setLoginTimeout(int seconds) throws SQLException
{
}
@Override
public int getLoginTimeout() throws SQLException
{
return 0;
}
// Main method to demonstrate usage of DataSourceFactory
public static void main(String[] args)
{
// Create an instance of DataSourceFactory
DataSource dataSource = new DataSourceFactory();
// SQL query to retrieve data from the "employees" table
String query = "SELECT * FROM employees WHERE dept = ?";
try (Connection connection = dataSource.getConnection();
PreparedStatement statement = connection.prepareStatement(query)) {
// Set parameter for the SQL query
statement.setString(1, "IT");
// Execute the query
try (ResultSet resultSet = statement.executeQuery()) {
// Iterate over the result set and print data
while (resultSet.next()) {
String name = resultSet.getString("name");
int age = resultSet.getInt("age");
System.out.println("Name: " + name + ", Age: " + age);
}
}
} catch (SQLException e) {
// Handle SQL exceptions
e.printStackTrace();
}
}
}
Explanation of the Code:
- DataSourceFactory Class is implement the javax.sql.DataSource interface. It provides the method for obtaining the connections of the database.
- getConnection Method is allowed to the getting a connections to the database with the specified URL, username and passwords.
- Main method is created the instance of the DataSourceFactory. It executes the SQL query to retrieve the data for IT department in the employees table in the database and it prints the results.
- Exception Handling is used to catch the SQL exceptions while occurring the query execution and print them.
Note: Make sure you should replace the connection parameters such as URL, username, password with your details of the database connections. And replace the SQL query and parameters names with the your query and parameter names.
Step 6: Run the code
- Right click on the java class file and select Run As > Java Application.
- After run the code, the output will be shown in the console window in your eclipse as shown below.
Output:
Below we can refer the console output image for better understanding.
Please Login to comment...