Materials in WebGL and p5.js
Last Updated :
17 Mar, 2021
In this article, we will learn how to apply different types of material in WebGL. Light reflects off objects differently depending on their angle of reflection as well as the material of the object. There are four types of materials in p5.js:
- Basic Material: It fills the geometry with the given color, but it is not affected by light. It is defined with the fill() method.
- Normal Material: It does not take any parameter and maps automatically to the RGB color space. It is defined with the normalMaterial() method.
- Ambient Material: It reflects only if there is the light of a particular color. It is defined with the ambientMaterial() method.
- Specular Material: It is the most realistic of all four materials. Specular is a technical way of describing a material that reflects light in a single direction. It is defined with the specularMaterial() method.
Example 1: Using the fill() method.
Javascript
let angle = 0.3;
function setup() {
createCanvas(600, 400, WEBGL);
}
function draw() {
background(250);
fill(200,0,255);
push();
rotateX(angle*0.3);
rotateY(angle*0.6);
rotateZ(angle*0.9);
torus(150, 30);
angle +=0.06;
pop();
}
|
Output:
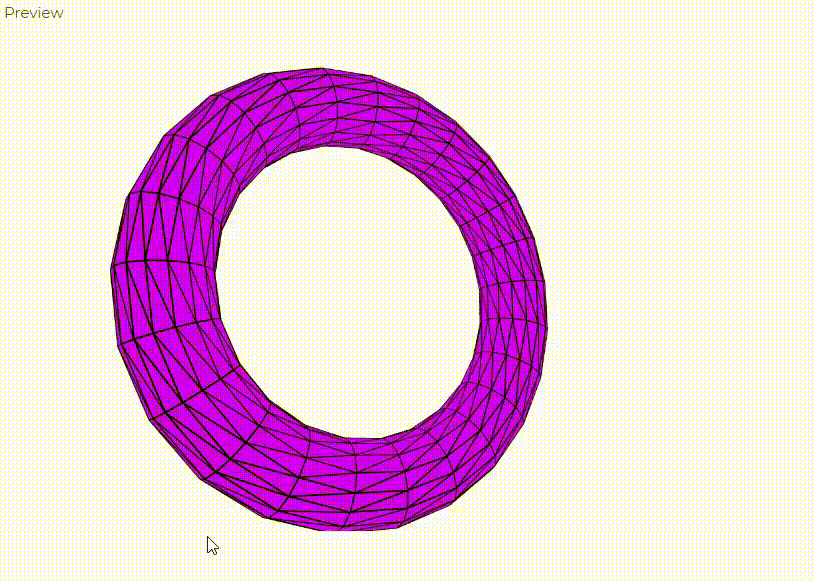
Example 2: Using the normalMaterial() method.
Javascript
let angle = 0.3;
function setup() {
createCanvas(600, 400, WEBGL);
}
function draw() {
background(250);
normalMaterial();
push();
rotateX(angle*0.3);
rotateY(angle*0.6);
rotateZ(angle*0.9);
torus(150, 30);
angle +=0.06;
pop();
}
|
Output:
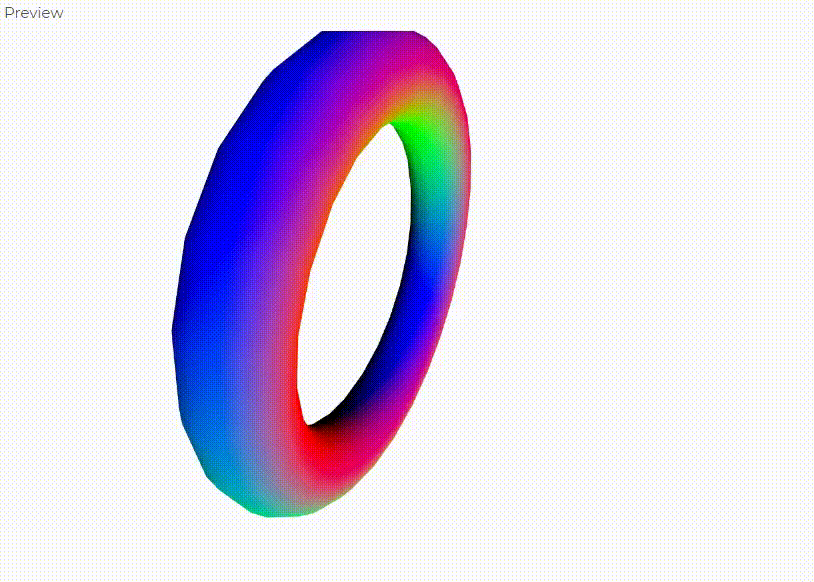
Example 3: Using ambientMaterial() when there is no light.
Javascript
let angle = 0.3;
function setup() {
createCanvas(600, 400, WEBGL);
}
function draw() {
background(250);
ambientMaterial(0,0,255);
push();
rotateX(angle*0.3);
rotateY(angle*0.6);
rotateZ(angle*0.9);
torus(150, 30);
angle +=0.06;
pop();
}
|
Output:
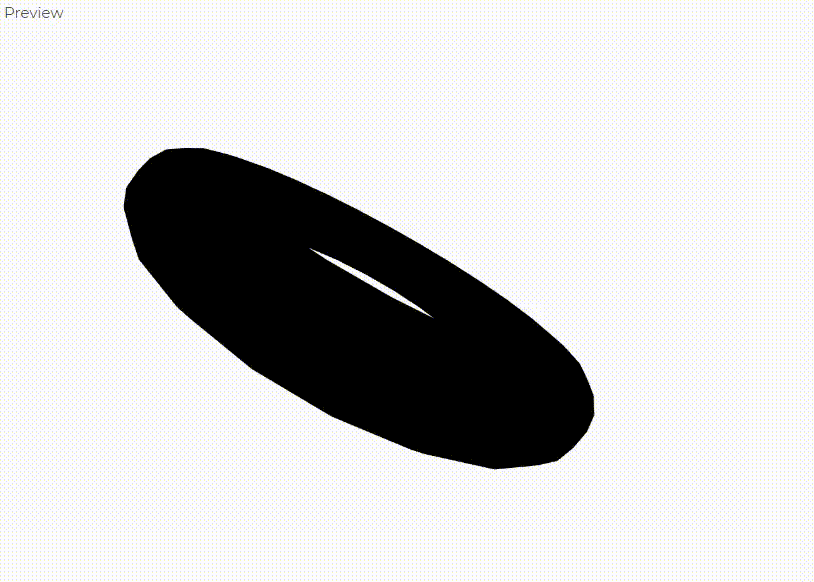
Example 4: Using ambientMaterial() when there is light that is being reflected.
Javascript
let angle = 0.3;
function setup() {
createCanvas(600, 400, WEBGL);
}
function draw() {
pointLight(0,0,255 ,200,-200,0)
background(250);
ambientMaterial(0,0,255);
push();
rotateX(angle*0.3);
rotateY(angle*0.6);
rotateZ(angle*0.9);
torus(150, 30);
angle +=0.06;
pop();
}
|
Output:
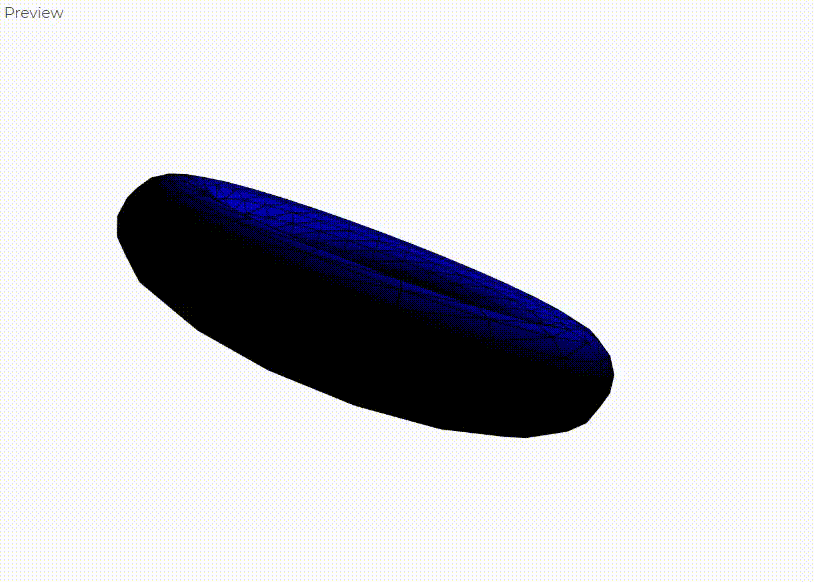
Example 4: Using specularMaterial() method.
Javascript
let angle = 0.3;
function setup() {
createCanvas(600, 400, WEBGL);
}
function draw() {
pointLight(255,255,0 ,200,-200,0)
background(250);
specularMaterial(250, 0, 0);
push();
rotateX(angle*0.3);
rotateY(angle*0.6);
rotateZ(angle*0.9);
torus(150, 30);
angle +=0.06;
pop();
}
|
Output:
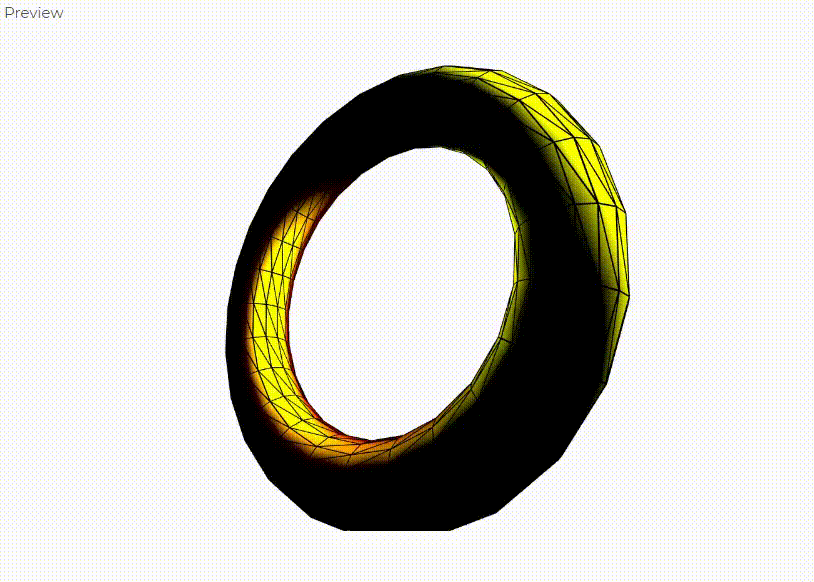
Share your thoughts in the comments
Please Login to comment...