Print Lines Starting with String in Linux
Last Updated :
17 Jun, 2021
Printing lines that contain a particular string at the beginning is quite annoying to manually deal with, so we can make use of bash to design a shell script. The most versatile and powerful tool for searching patterns or lines is by using grep, awk, or sed to find the most efficient solution to the problem.
The first thing to get started with is the file in which the string is to be searched and also the string. Both of the parameters will be inputted from the user. After that, we can use any one of the three tools grep, sed or awk. The concept is the same, we need to find the string only at the beginning of a line using regex and print the lines depending on the tool to be used.
User input
We will input the file name and string from the user. We will use the read command and pass in the -p argument to prompt the user a text to display what he/she should input. We will store the input in the appropriate variable names.
#!/bin/bash
read -p “Enter the file name : ” file
read -p “Enter the string to search for in the file : ” str
Searching and Printing Lines
The following are the three tools used to perform the operation of finding and printing lines that have a string input from the user. Anyone can be used as per user choice and requirements.
Method 1: Using GREP Command
The grep command is quite useful for finding patterns in the file or strings in the line. We will be using the simple grep command that searches for the word at the beginning of the file using the ^ operator and prints the line from the file. This is quite straightforward to understand if you are familiar with the grep command in Linux. The grep command will simply search for the input string/word from the file and print the line in which the string occurs only at the beginning. The last argument, which is “grep -v grep || true” only returns 0 if the grep didn’t find any match. This will not show the annoying error of shell return 1, it will not print anything and the user will understand that no line started with that string in the file.
#!/bin/bash
read -p "Enter the file name : " file
read -p "Enter the string to search for in the file : " str
grep "^$str" $file | { grep -v grep || true; }
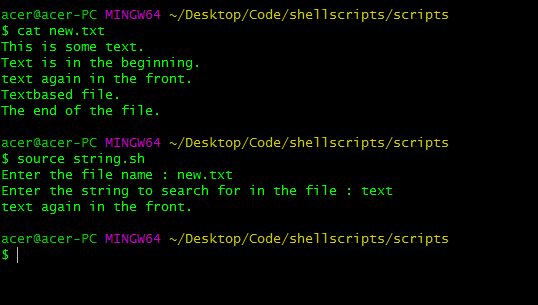
using the grep command to search for strings at the start of lines.
The grep command is also a bit flexible as it allows us to print the line numbers as well. We can print the line numbers by adding some arguments to the above command. The -n argument after grep will print the line number in which the string is found in the file.
#!/bin/bash
read -p "Enter the file name : " file
read -p "Enter the string to search for in the file : " str
grep -n "^$str" $file | { grep -v grep || true; }
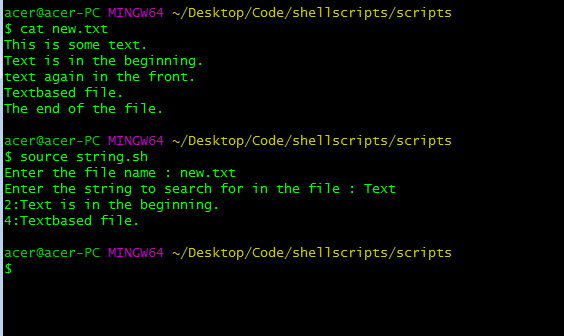
using the grep command to search for strings at the start of lines and print line numbers.
Both the cases in grep will be case-sensitive. To make the search case insensitive, you can add in the argument -i just after the grep like,
grep -i "^$str" $file | { grep -v grep || true; }
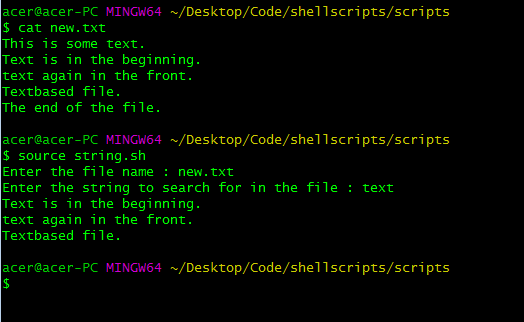
using grep command to search case insensitive strings at the start of lines.
Method 2: Using SED Command
The sed command is different than the grep command as it is a stream editor and not a command to just pass in arguments. We need to use -n so that the command doesn’t print everything from the file provided in the last argument of the command below. The following regex will search for the string variable and print all the matched lines. And the result will be compact output. The regex used is simple and similar to grep as it matches the string only at the beginning of the line using the ^ operator and backslashes mean the expression is a regex. The p argument will print the lines which match the regex. Both the cases in grep will be case-sensitive.
#!/bin/bash
read -p "Enter the file name : " file
read -p "Enter the string to search for in the file : " str
sed -n "/^$str/p" $file
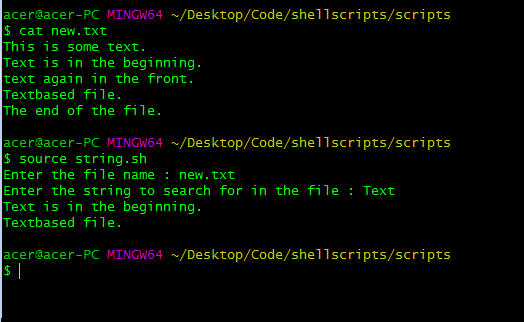
using sed command to search for strings at the start of lines.
The gnu sed doesn’t support case-insensitive searches or matches. Still, you can use Perl to add case-insensitive matches.
Method 3: Using AWK Command
The regex for the AWK command is also the same and does the same thing in its own command style. The regex is almost similar to the sed command, which searches for the string variable, in the beginning, using the ^ operator and then simply prints the matched lines from the command. Both the cases in grep will be case-sensitive.
#!/bin/bash
read -p "Enter the file name : " file
read -p "Enter the string to search for in the file : " str
awk "/^$str/{print}" $file
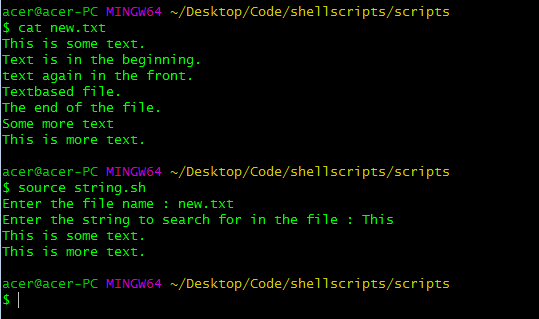
using the awk command to search for strings at the start of lines.
To make the search in awk case insensitive, you can make some changes to the same command.
awk "BEGIN {IGNORECASE = 1} /^$str/{print}" $file
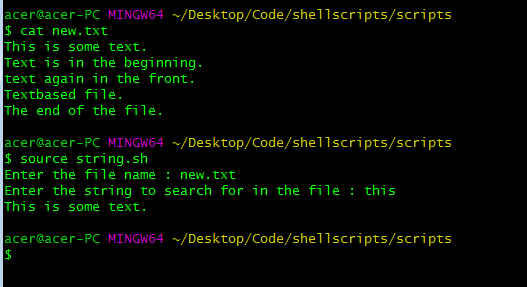
using the awk command to search for case insensitive strings at the start of lines.
The awk command has some inbuilt functions which set the arguments as default to case sensitive, we can change the properties of the awk commands.
Share your thoughts in the comments
Please Login to comment...